How to Get a Word Count for Any Folder in Your Obsidian Vault
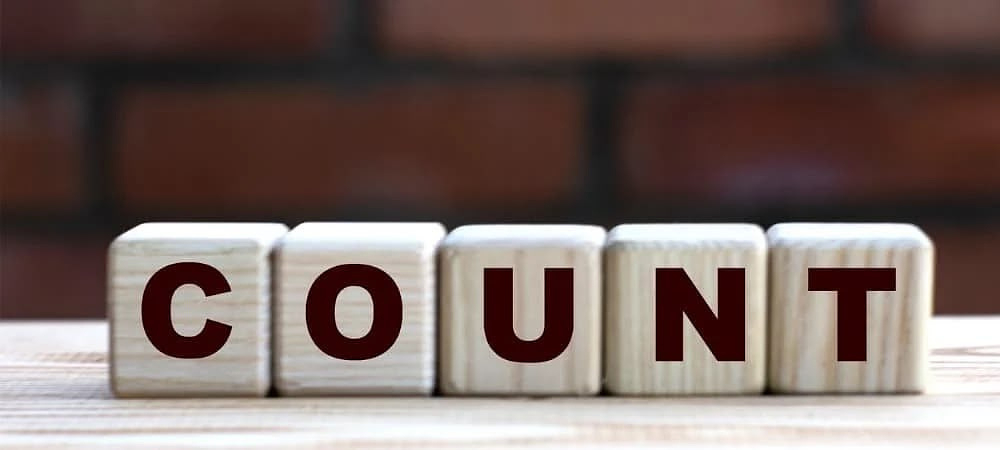
A Python script that will count the words in a folder of markdown files. #Obsidian #ObsidianMD #PKM
I use Obsidian to write a minimum of three blog posts every day as well as technical documents for my job. Of course, I also compose and edit notes in it too. At the end of 2024, I was curious to see how many words I'd written on each blog during the year. Unfortunately, I could not find a plugin that could do this, but I suspected that Python probably could. After working on it for a while with the help of Google Gemini, I had an easy to run script that would work on any folder in my vault. If you have any Python experience, you won't find this difficult at all to use. The only edit you need to make is for the path of the folder you want to evaluate. Just save this in a text editor like BBEdit with a .py extension. Change the permissions on it using chmod and it will be ready to run.
chmod +x pythonScript.py
The Script
\#!/usr/bin/env python3 import os def count_words_in_markdown(filepath): """Counts the number of words in a markdown file. Args: filepath: Path to the markdown file. Returns: The number of words in the file. """ with open(filepath, 'r', encoding='utf-8') as f: content = f.read() \# Simple word counting by splitting on whitespace words = content.split() return len(words) def count_words_in_directory(directory): """Counts the total number of words in all markdown files within a directory. Args: directory: Path to the directory containing markdown files. Returns: The total word count across all markdown files. """ total_words = 0 for filename in os.listdir(directory): if filename.endswith(".md"): filepath = os.path.join(directory, filename) total_words += count_words_in_markdown(filepath) return total_words if __name__ == "__main__": directory_to_search = "PUT THE PATH TO A FOLDER HERE" \# Replace with your directory total_word_count = count_words_in_directory(directory_to_search) print(f"Total words in markdown files: {total_word_count}")